HTML5 Canvas는 웹 브라우저 내에서 멋진 대화형 그래픽을 만들 수 있는 강력한 도구입니다. 이 글에서는 HTML5 Canvas 기능 대화형 그래픽을 만드는 방법에 대한 실제 예시를 보여줍니다. 어렵지 않으니 코드를 보면서 차근차근 글을 읽어 보시고, 따라오시면 됩니다. 자 그럼 시작해 볼까요?
[목차]
캔버스 설정
HTML5 Canvas를 사용하려면 웹 페이지 내에서 <canvas> 태그를 설정해야 합니다. 이 태그는 JavaScript를 통해 스타일을 지정하고 제어할 수 있는 캔버스 영역을 정의합니다.
<canvas id="myCanvas" width="800" height="600"></canvas>
기본 도형 그리기
캔버스 API를 사용하면 선, 사각형, 원, 다각형 등 다양한 도형을 그릴 수 있습니다. 다음은 몇 가지 기본적인 예시입니다.
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
// 선 그리기
ctx.beginPath();
ctx.moveTo(50, 50);
ctx.lineTo(200, 50);
ctx.strokeStyle = "red";
ctx.lineWidth = 2;
ctx.stroke();
// 사각형 그리기
ctx.fillStyle = "blue";
ctx.fillRect(50, 100, 150, 100);
// 원 그리기
ctx.beginPath();
ctx.arc(400, 200, 50, 0, 2 * Math.PI);
ctx.fillStyle = "green";
ctx.fill();
애니메이션 만들기
HTML5 Canvas를 사용하면 캔버스 콘텐츠를 지속적으로 업데이트하여 매력적인 애니메이션을 만들 수 있습니다. 이는 requestAnimationFrame 함수를 사용하고 각 프레임 내에서 캔버스를 업데이트하여 달성됩니다.
function animate() {
// 캔버스 지우기
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 위치 업데이트
x += dx;
y += dy;
// 새 위치에서 모양 그리기
ctx.beginPath();
ctx.arc(x, y, 20, 0, 2 * Math.PI);
ctx.fillStyle = "orange";
ctx.fill();
// 경계 확인 및 방향 전환
if (x + dx > canvas.width || x + dx < 0) {
dx = -dx;
}
if (y + dy > canvas.height || y + dy < 0) {
dy = -dy;
}
// 다음 애니메이션 프레임 요청
requestAnimationFrame(animate);
}
// 초기 위치 및 속도
let x = 100;
let y = 100;
let dx = 2;
let dy = 2;
// 애니메이션 시작
animate();
사용자 상호 작용 처리
HTML5 Canvas를 사용하면 마우스 이동 및 클릭과 같은 사용자 상호 작용을 캡처하여 대화형 그래픽을 만들 수 있습니다. 다음은 사용자가 모양을 클릭할 때 모양의 색상을 변경하는 예입니다.
// 캔버스에 클릭 이벤트 리스너 추가
canvas.addEventListener("click", function (event) {
const rect = canvas.getBoundingClientRect();
const mouseX = event.clientX - rect.left;
const mouseY = event.clientY - rect.top;
if (ctx.isPointInPath(mouseX, mouseY)) {
ctx.fillStyle = getRandomColor();
}
});
// 랜덤 색상 생성
function getRandomColor() {
const letters = "0123456789ABCDEF";
let color = "#";
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
전체코드 보기
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 | <!DOCTYPE html> <html> <head> <title>HTML5 Canvas: Creating Interactive Graphics</title> <style> canvas { border: 1px solid #ccc; } </style> </head> <body> <h1>HTML5 Canvas: Creating Interactive Graphics</h1> <canvas id=“myCanvas” width=“800” height=“600”></canvas> <script> // Drawing Basic Shapes const canvas = document.getElementById(“myCanvas”); const ctx = canvas.getContext(“2d”); // Draw a line ctx.beginPath(); ctx.moveTo(50, 50); ctx.lineTo(200, 50); ctx.strokeStyle = “red”; ctx.lineWidth = 2; ctx.stroke(); // Draw a rectangle ctx.fillStyle = “blue”; ctx.fillRect(50, 100, 150, 100); // Draw a circle ctx.beginPath(); ctx.arc(400, 200, 50, 0, 2 * Math.PI); ctx.fillStyle = “green”; ctx.fill(); // Creating Animations let x = 100; let y = 100; let dx = 2; let dy = 2; function animate() { ctx.clearRect(0, 0, canvas.width, canvas.height); x += dx; y += dy; ctx.beginPath(); ctx.arc(x, y, 20, 0, 2 * Math.PI); ctx.fillStyle = “orange”; ctx.fill(); if (x + dx > canvas.width || x + dx < 0) { dx = –dx; } if (y + dy > canvas.height || y + dy < 0) { dy = –dy; } requestAnimationFrame(animate); } animate(); // Handling User Interactions canvas.addEventListener(“click”, function (event) { const rect = canvas.getBoundingClientRect(); const mouseX = event.clientX – rect.left; const mouseY = event.clientY – rect.top; if (ctx.isPointInPath(mouseX, mouseY)) { ctx.fillStyle = getRandomColor(); } }); function getRandomColor() { const letters = “0123456789ABCDEF”; let color = “#”; for (let i = 0; i < 6; i++) { color += letters[Math.floor(Math.random() * 16)]; } return color; } </script> </body> </html> | cs |
실행화면
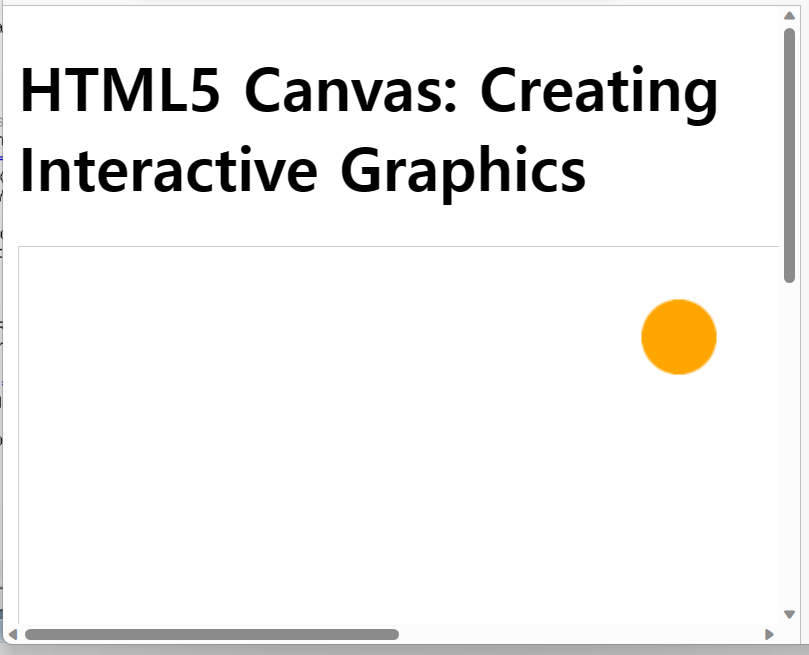
HTML5 Canvas: Creating Interactive Graphics
결론
HTML5 Canvas를 사용하면 웹 브라우저 내에서 창의적인 대화형 그래픽을 만들 수 있습니다. 이 글에서 다룬 예시와 개념을 통해 HTML5 Canvas의 가능성을 탐색할 수 있는 견고한 기반을 갖추게 되었습니다. 이제 HTML5 Canvas를 사용하여 창의력을 발휘하고 매력적인 대화형 그래픽을 사용하시기 바랍니다.
[관련글]
파이썬 웹크롤링(crawling), PyQt6, BeautifulSoup