CSS(Cascading Style Sheets)는 HTML 문서를 시각적으로 매력적인 웹 페이지로 변환하는 중추적인 역할을 합니다. CSS를 사용하면 개발자가 웹 콘텐츠의 프레젠테이션 및 레이아웃을 제어하여 멋진 디자인과 원활한 사용자 경험을 만들 수 있습니다. 이 글에서는 CSS 웹 페이지 스타일 변경 및 수정에 대해 알아보겠습니다.
[목차]
선택기 및 규칙 세트
CSS는 선택기를 사용하여 HTML 요소에 스타일을 적용하여 작동합니다. 선택기는 특정 요소 또는 요소 그룹을 대상으로 하므로 사용자 정의 스타일을 정의할 수 있습니다. 규칙 세트는 적용할 스타일을 정의하는 중괄호로 묶인 선언 세트와 선택기로 구성됩니다.
/* Selector targets an element with the 'class' attribute */
.my-class {
color: blue;
font-size: 18px;
}
색상 및 타이포그래피
CSS는 웹 페이지에서 색상과 타이포그래피를 사용자 정의할 수 있는 다양한 옵션을 제공합니다. CSS를 사용하면 글꼴 패밀리, 크기, 두께 및 스타일을 제어하고 텍스트 색상과 배경을 조작할 수 있습니다.
/* Styling heading elements */
h1 {
font-size: 32px;
font-weight: bold;
color: #333;
}
/* Changing background color */
body {
background-color: #f4f4f4;
}
/* Adding custom fonts */
body {
font-family: 'Roboto', sans-serif;
}
박스 모델 및 레이아웃
상자 모델을 이해하는 것은 잘 구성된 웹 페이지 레이아웃을 만드는 데 필수적입니다. 각 HTML 요소는 너비, 높이, 패딩, 테두리 및 여백과 같은 속성이 있는 직사각형 상자로 간주됩니다. 이러한 속성을 조작하여 간격, 정렬 및 전체 레이아웃을 제어할 수 있습니다.
/* Setting box dimensions */
.box {
width: 200px;
height: 200px;
}
/* Adjusting padding and margins */
.box {
padding: 20px;
margin: 10px;
}
/* Adding borders */
.box {
border: 2px solid #ccc;
}
플렉스박스와 그리드
CSS는 Flexbox와 CSS Grid라는 두 가지 강력한 레이아웃 시스템을 제공합니다. 이는 컨테이너 내에서 요소를 배치하고 정렬하는 보다 진보되고 유연한 접근 방식을 제공합니다. Flexbox는 1차원 레이아웃에 초점을 맞추는 반면 CSS Grid는 2차원 레이아웃을 허용합니다.
/* Flexbox layout */
.container {
display: flex;
justify-content: center;
align-items: center;
}
/* CSS Grid layout */
.container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-gap: 20px;
}
전환 및 애니메이션
CSS 전환 및 애니메이션을 사용하면 웹 페이지에 동적 효과와 상호 작용을 추가할 수 있습니다. CSS를 사용하면 속성 값 간에 부드러운 전환을 생성하고 키프레임을 사용하여 복잡한 애니메이션을 정의할 수 있습니다.
/* Transition on hover */
.button {
background-color: #333;
color: #fff;
transition: background-color 0.3s;
}
.button:hover {
background-color: #f44336;
}
/* Keyframe animation */
@keyframes spin {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
.spinner {
animation: spin 2s linear infinite;
}
전체코드 보기
이 코드를 HTML 파일에 저장하고 웹 브라우저에서 열어서 CSS 스타일이 적용되는 것을 확인합니다. 웹 페이지에는 색상, 타이포그래피, Flexbox 또는 CSS Grid를 사용한 레이아웃, 전환 및 애니메이션을 포함하여 CSS 스타일 지정의 다양한 예가 표시됩니다
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 | <!DOCTYPE html> <html> <head> <title>Introduction to CSS: Web Page Styling</title> <style> /* CSS styles go here */ .my-class { color: blue; font-size: 18px; } h1 { font-size: 32px; font-weight: bold; color: #333; } body { background-color: #f4f4f4; font-family: ‘Roboto’, sans-serif; } .box { width: 200px; height: 200px; padding: 20px; margin: 10px; border: 2px solid #ccc; } .container { display: flex; justify-content: center; align-items: center; } /* Uncomment the following CSS for CSS Grid layout */ /* .container { display: grid; grid-template-columns: 1fr 1fr 1fr; grid-gap: 20px; } */ .button { background-color: #333; color: #fff; transition: background-color 0.3s; } .button:hover { background-color: #f44336; } @keyframes spin { 0% { transform: rotate(0deg); } 100% { transform: rotate(360deg); } } .spinner { animation: spin 2s linear infinite; } </style> </head> <body> <h1>Introduction to CSS: Web Page Styling</h1> <div class=“my-class”> This is an example of styling using a class. </div> <h2>Color and Typography</h2> <p>This is a paragraph with custom font and color.</p> <div class=“box”> This is a box element with specific dimensions, padding, margin, and border. </div> <h2>Layout with Flexbox</h2> <div class=“container”> <div>Item 1</div> <div>Item 2</div> <div>Item 3</div> </div> <!– Uncomment the following HTML for CSS Grid layout –> <!– <h2>Layout with CSS Grid</h2> <div class=”container”> <div>Item 1</div> <div>Item 2</div> <div>Item 3</div> </div> –> <h2>Transitions and Animations</h2> <button class=“button”>Hover Me</button> <div class=“spinner”>This element spins!</div> <script> // JavaScript code can be added here if needed </script> </body> </html> | cs |
실행화면
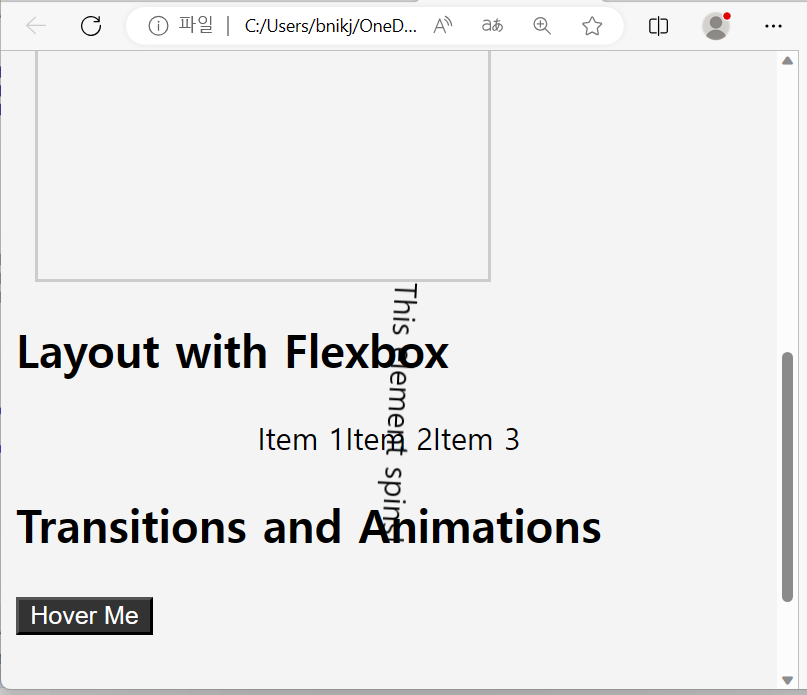
Introduction to CSS: Web Page Styling
Color and Typography
This is a paragraph with custom font and color.
Layout with Flexbox
Transitions and Animations
결론 및 의견
CSS는 웹 개발자가 웹 페이지를 시각적으로 매력적이고 매력적인 경험으로 변환할 수 있게 해주는 강력한 도구입니다. CSS의 기능을 활용하여 색상, 타이포그래피, 레이아웃, 전환 및 애니메이션을 제어하여 사용자를 사로잡는 멋진 디자인을 만들 수 있습니다. 지금까지 CSS 웹 페이지 스타일 수정 대해 알아봤습니다.
[관련글]